In Python programming, efficiency and simplicity often go hand in hand. The tools and functions provided within this language offer straightforward solutions to potentially complex problems, making tasks like array creation more accessible.
Using the range() Function
Creating an array of numbers in Python is simple, thanks to the range()
function. This built-in function generates a sequence of numbers, streamlining array creation.
The range()
function requires a start and stop value, with an optional step parameter to specify the increment between each number in the sequence.
Here's how to use it:
# Create an array of numbers from 1 to 10
array_of_numbers = range(1, 11)
In this example, 1
is the starting point, and 11
is just after the end point since the stop value isn't included. The function returns a range object. To see the numbers, convert this object into a list:
# Convert range object into a list
list_of_numbers = list(array_of_numbers)
print(list_of_numbers)
This will output the array of numbers from 1 to 10:
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
The optional step parameter is useful for creating sequences with specific patterns. For example, to create an array of every other number from 1 to 10:
# Create an array of every other number from 1 to 10
array_of_even_numbers = range(1, 11, 2)
print(list(array_of_even_numbers))
This prints out:
[1, 3, 5, 7, 9]
Here, 2
is the step, ensuring the function skips every other number.
The range()
function provides an efficient way to generate numbers within a specified interval in Python. This tool, used alone or as part of list comprehensions, offers a neat way to create arrays without requiring complex loops or additional libraries.
List Comprehensions for Array Creation
Beyond the range()
function, a powerful feature of Python for generating arrays is list comprehension. List comprehensions provide a concise way to create lists or arrays, making code more readable and efficient, especially when dealing with numerical sequences or transformations.
List comprehension is a one-liner that generates a list in Python. It follows the format [expression for item in iterable]
, making it straightforward to understand and implement. For example, to create an array of numbers from 1 to N:
N = 10
numbers = [i for i in range(1, N+1)]
print(numbers)
This code snippet generates a list of numbers from 1 to 10. This approach is cleaner and often executes faster than conventional for loops, particularly for larger values of N.
List comprehensions also allow for incorporating conditions. For example, to create an array of only even numbers:
N = 10
even_numbers = [i for i in range(1, N+1) if i % 2 == 0]
print(even_numbers)
Here, i % 2 == 0
checks if the number is even, and only then does it get added to the resulting array. This demonstrates how list comprehensions support conditional logic efficiently without requiring additional loop constructs or filtering steps post array creation.
Compared to traditional methods of array creation like appending to a list in a loop, list comprehensions are syntactically pleasing and reduce the chance of errors, such as accidentally manipulating a global variable within a loop. This makes code safer and more reliable.
List comprehensions embody Python's philosophy of simplicity and elegance. They offer an efficient way to create arrays and allow for nuanced manipulations within those arrays through a cleaner and more readable syntax.
Employing list comprehensions can greatly enhance coding efficiency compared to traditional array creation methods. With their ability to integrate conditional logic and execute operations within a single readable line, they encourage cleaner code with the same or even improved performance.
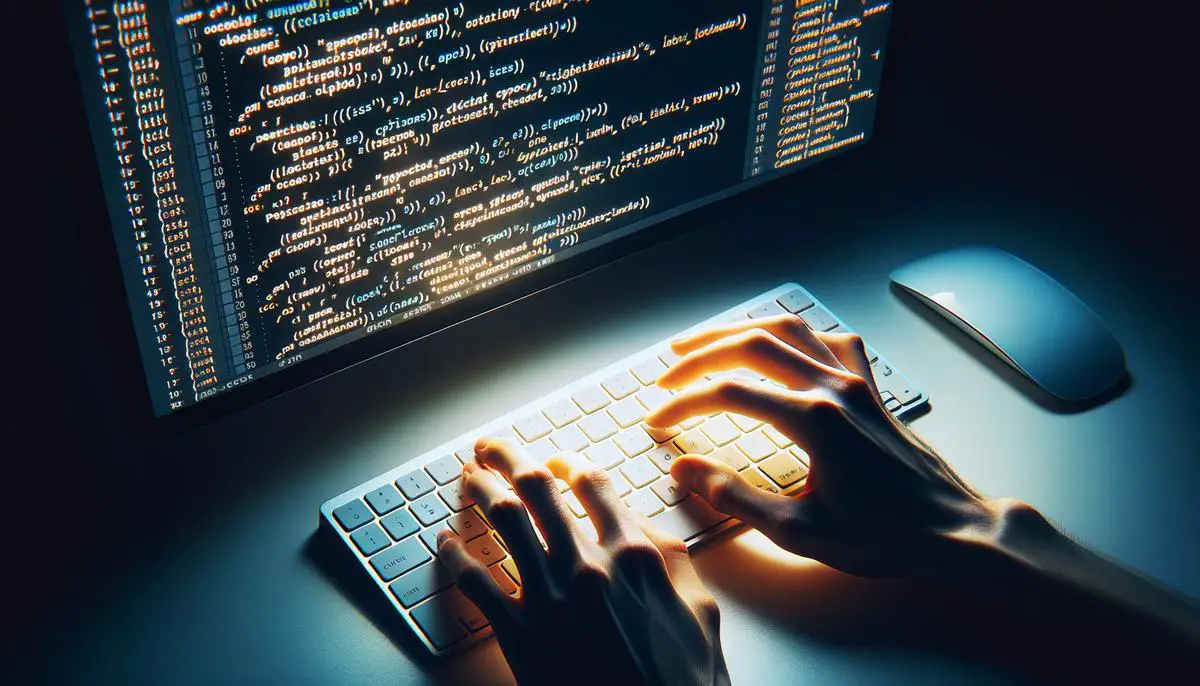
Utilizing NumPy for Large Arrays
While list comprehensions and the range()
function in Python offer elegance and simplicity, NumPy emerges as a stronger option, especially when dealing with large numeric arrays.
Handling vast numerical arrays using simple Python lists or relying entirely on the range()
function with loops works well for small to medium-sized tasks. However, as the scale increases, the time complexity and memory consumption can become significant. That's where NumPy comes into play, offering efficiency and scalability.
NumPy, short for Numerical Python, is a library designed for numerical computations. It introduces the numpy array, a grid of values all of the same type, significantly optimized for large-sized operations. NumPy allows for performing complex mathematical operations efficiently and with less code compared to multi-layered loops and lists in plain Python.
One of the key features of NumPy is its speed. NumPy arrays take advantage of C language efficiency since the library is built on it. This integration allows operations to bypass the interpretation layer of Python, reducing operation time and memory usage drastically compared to lists.
- NumPy extends its functionality with functions for:
- Statistical analysis
- Linear algebra
- Random number generation
This makes it a comprehensive solution for numerical computations in Python. It also integrates seamlessly with databases and external libraries or tools.
NumPy arrays come with meta-information like 'shape' and 'dimension,' providing an additional layer of ease to manipulate large datasets. Reshaping or slicing hefty data arrays becomes straightforward with NumPy.
While diving into NumPy might seem intimidating initially due to its vast functionality, the return on investment is substantial. Whether processing images, optimizing algorithms, or crunching large datasets for insights, NumPy stands as a beacon of efficiency, keeping code clean and operation times low.
NumPy transforms how we approach computations in Python, enriching our toolkit with its broad array of features. Its design philosophy is to streamline complex numeric computations, making them more accessible and less time-consuming. Its efficiency, seamless operation, and breadth of features make NumPy the preferred choice among Python enthusiasts for numerical computations.
When faced with large arrays and the need to perform computations that seem daunting with lists or the range()
function alone, NumPy is a powerful tool. It simplifies high-level mathematical operations, making numeric computations feel like second nature within Python's environment.
NumPy's utility in handling large numeric arrays cannot be overstated. It exemplifies Python's capability to simplify complex numerical computations, making them more manageable and less time-consuming. This efficiency is paramount for developers working with extensive datasets or requiring high-level mathematical operations. NumPy stands as a testament to Python's power in numerical computing, offering an indispensable tool for those looking to enhance their programming efficacy.
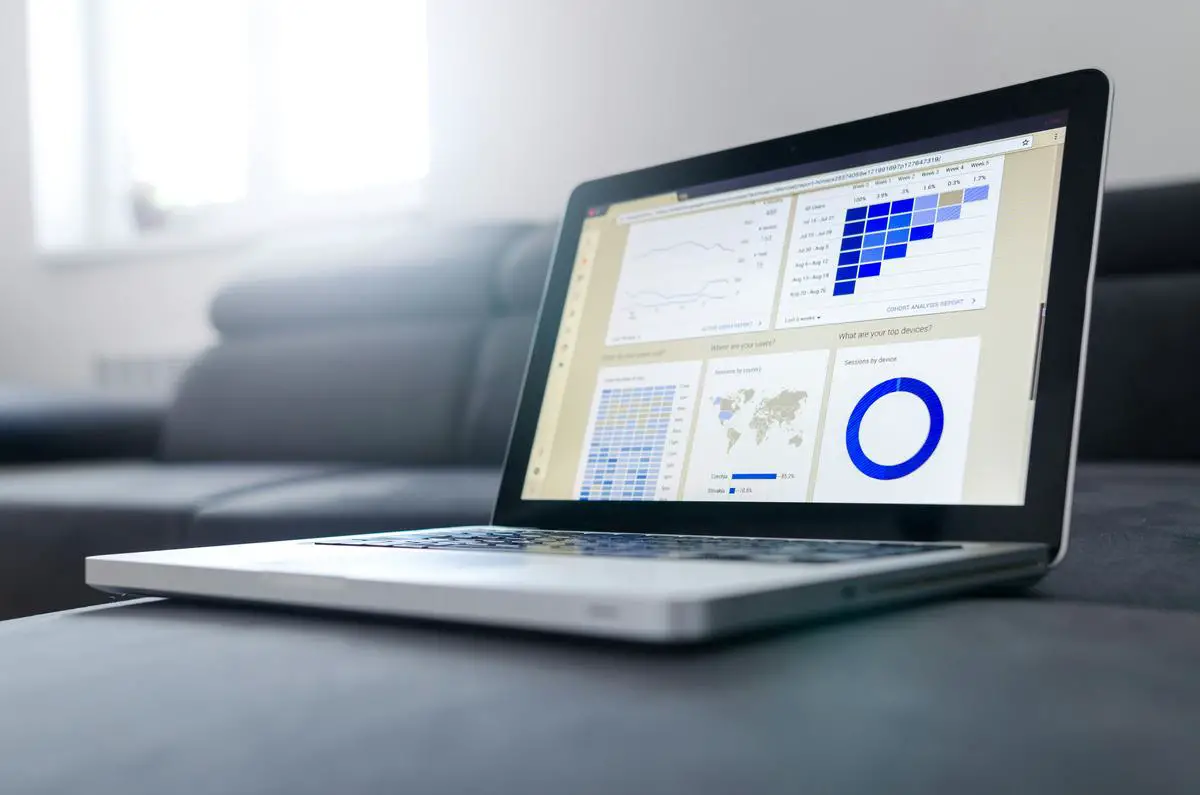
- Van Rossum G, Drake FL. Python 3 Reference Manual. Scotts Valley, CA: CreateSpace; 2009.
- McKinney W. Python for Data Analysis: Data Wrangling with Pandas, NumPy, and IPython. Sebastopol, CA: O'Reilly Media; 2017.
- Oliphant TE. A Guide to NumPy. USA: Trelgol Publishing; 2006.
Revolutionize your content with Writio, the AI writing platform. This article was crafted by Writio.