In an era dominated by cloud computing and an ever-growing demand for automation, the understanding and effective usage of Amazon Web Services’ Command Line Interface (AWS CLI) has become a sought-after skill. The AWS CLI provides a unified tool to manage and interact with all of Amazon’s services. Whether you are a developer, system administrator, or simply a tech enthusiast hoping to understand and leverage the power of AWS CLI, this in-depth exploration will set you on the path to mastery. From installing and configuring your environment, diving deep into the fundamentals of AWS CLI, learning to write and run scripts with precision, to applying these skills in real-world scenarios, we will delve into all the crucial aspects.
Understanding AWS CLI
Step 1: Installing and Updating AWS CLI
The first step to diving deep into AWS CLI (Command Line Interface) is installing it. Install the AWS CLI on Mac, Linux, or Windows using the official AWS instructions. These may vary by operating system, but typically the AWS CLI can be installed using pip, the Python install package. Installation includes this version and a subsequent version that includes additional functionality. Regular updates using pip are also essential for security and to access new features.
Step 2: Configuring AWS CLI
After installing AWS CLI, you’ll need to set up your environment. Use the command ‘aws configure’ to start the process. This will prompt you to enter your Access Key ID and Secret Access Key, which link the CLI to your AWS account. You’ll also have the opportunity to set a default region: typically, this should be the region that most of your resources are in, or the region that you interact with the most. Lastly, set your default output format, which controls how information is displayed in the terminal.
Step 3: Learning Basic AWS CLI Commands
The ‘aws’ command is at the root of all interaction with AWS CLI. Help commands are an easy way to find more information about any command or operation. For example, ‘aws s3 help’ will bring up a list of all commands related to S3. Other fundamental commands include ‘aws s3 cp’ to copy files to and from S3 buckets, or ‘aws ec2 describe-instances’ to get information about EC2 instances.
Step 4: Exploring AWS CLI With Different Services
AWS CLI can interact with nearly every AWS service. However, each service requires specific commands to operate. For instance, AWS S3 involves commands for creating, listing, and deleting buckets as well as uploading, downloading, and removing objects in the buckets. On the other hand, AWS EC2 commands include those for launching, listing, and terminating instances. Other services like Lambda, DynamoDB, SQS, and more also have their unique commands.
Step 5: Authenticating AWS CLI
Authentication to AWS CLI is crucial for secure and correct access to your AWS account services. This process involves creating IAM users, roles, and establishing necessary access policies in your AWS Management Console. After that, you generate security credentials like Access Key ID and Secret Access Key for your IAM users. You then use these keys to run the ‘aws configure’ command, which authenticates your AWS CLI to AWS Account services. This authentication allows AWS CLI to access and manage the specified services as per the established policies.
Learning the AWS CLI scripting is about practicing with actual AWS services. Understand the basic commands, explore the unique commands for different services, configure, and authenticate. Over time, you will learn how to automate and manage AWS services more efficiently using the AWS CLI.
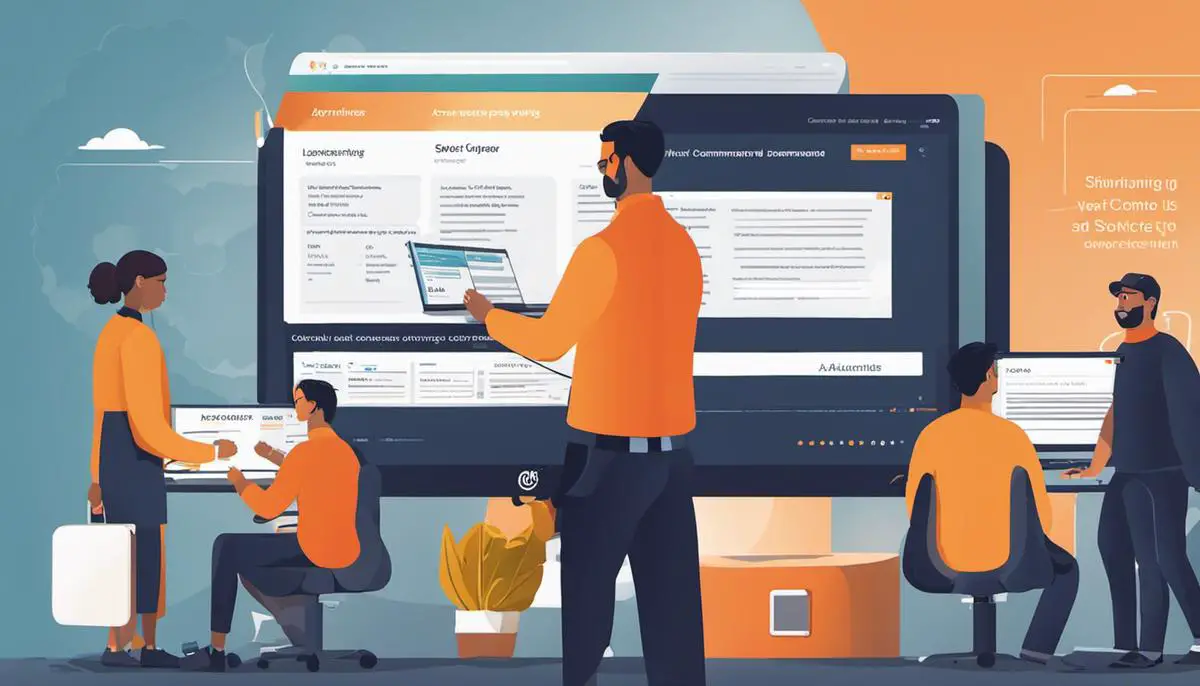
AWS CLI Scripting Basics
Installation of AWS CLI
First make sure you have AWS CLI (Command Line Interface) installed on your system. You can download it from the AWS website or you can install it using package managers like pip for Python.
Setting Up AWS CLI
After you have AWS CLI installed, you need to configure it to work with your AWS account. Use the command aws configure
to set your Access Key, Secret Access Key, region and output format.
Basics of AWS CLI Scripting
Start with familiarizing yourself with AWS CLI commands structure. All commands follow a pattern: aws [command] [sub-command] [options and parameters]
. For example, to list all your S3 buckets you would use the command: aws s3 ls
You can also run AWS CLI commands from within a shell script. To do this, simply include the AWS CLI command you want to run within the script.
For instance, if you’re using a bash script, it would look something like this:
#!/bin/bash
aws s3 ls
Dealing with Output
AWS CLI commands return output in a structured format either text, table, or JSON. You can use tools like jq to parse JSON output in the command line or you can use the --query
option in AWS CLI to filter the results.
Example,
#!/bin/bash
aws ec2 describe-instances --query 'Reservations[].Instances[].{ID: InstanceId, Type: InstanceType, State: State.Name}'
Prototyping Command Line Options
When writing scripts in AWS CLI, the --dry-run
option is handy. It makes AWS CLI attempt a dry-run execution of any write operations, meaning no changes are actually made, allowing you to test your script before running it for real.
Error Handling and Debugging
When scripting with the CLI, use the --debug
option to print debugging information. This helps when troubleshooting issues with your commands or scripts. It’s also generally a good practice to include error handling in your scripts.
Remember to always test scripts with non-destructive actions before scheduling or deploying them. Regularly check and review AWS CloudTrail logs for auditing and review.
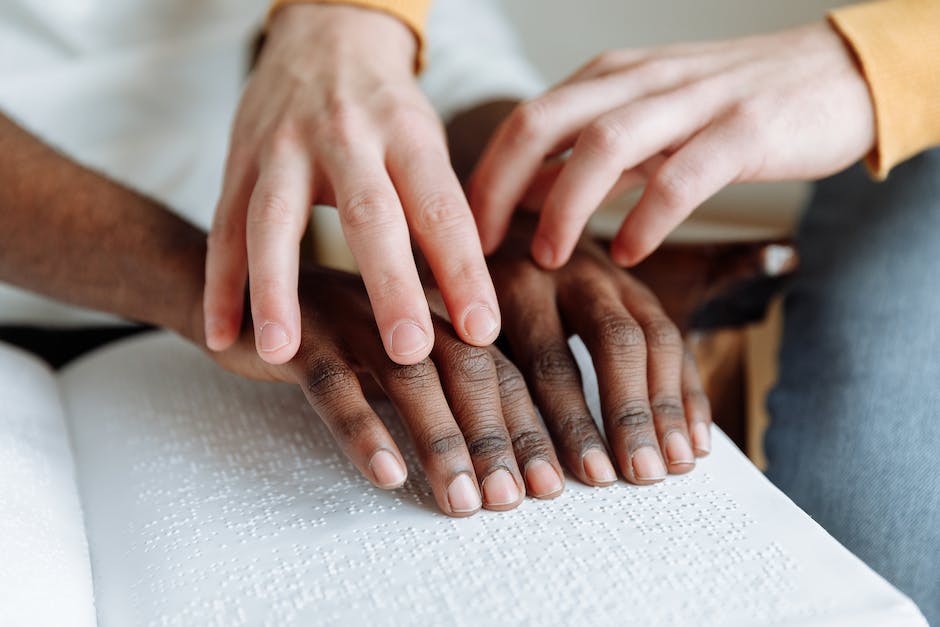
Advanced AWS CLI Scripting
Getting Familiar with AWS CLI Scripting
AWS Command Line Interface (CLI) scripting allows you to interact with various AWS services directly from your terminal. You can initiate multiple AWS service commands from a single script, combining the power of different services to achieve desired functionality.
Writing AWS CLI Scripts
To write AWS CLI scripts, you’ll need basics of scripting language like bash or Python. A typical script involves initiating an AWS API command, processing the response, and implementing some decision-making capability based on that response.
Example in bash:
#!/bin/bash
output=$(aws ec2 describe-instances --region us-west-2)
name=$(echo $output | jq -r '.Reservations[].Instances[].Tags[] | select(.Key=="Name") | .Value')
echo $name
This script communicates with the AWS EC2 service to retrieve information about instances in the us-west-2
region. The jq
tool is used here to parse JSON output to extract instance names.
Managing Errors in AWS CLI Scripts
A well-crafted AWS CLI script should contain provisions for handling errors. Errors are frequently returned in AWS CLI as part of the response object, separate from the normal output. You can identify and handle these using conditional logic in the script.
For instance, you might use an ‘if’ statement to check if an error object exists in the returned output and then handle it accordingly.
Improving Performance of AWS CLI Scripts
You can improve the performance of your AWS CLI scripts by applying certain best practices:
- Optimizing script logic: Avoid unnecessary loops, function calls, or redundant logic that can slow down the script’s execution.
- Implementing batch processing: When dealing with large amounts of data or resources, implement batch processing to increase efficiency.
- Limiting the data retrieved: You can limit the output to just the information you need by using the
--query
option in your AWS commands. This reduces network bandwidth and processing time.
Implementing Security Practices
Consider security while writing AWS CLI scripts. Always avoid hardcoding AWS credentials in your scripts. Instead, you can use AWS Identity and Access Management (IAM) roles to provide necessary permissions to AWS services. Another option is to store AWS credentials in a configuration file or use environment variables.
Mastering Advanced Topics
To further enhance your skills in AWS CLI scripting, learn about advanced topics such as:
- Integrating with AWS Lambda to execute scripts in response to events.
- Utilizing AWS Step Functions to coordinate multiple AWS services into serverless workflows.
- Implementing AWS CloudFormation to model and provision your AWS resources using templates.
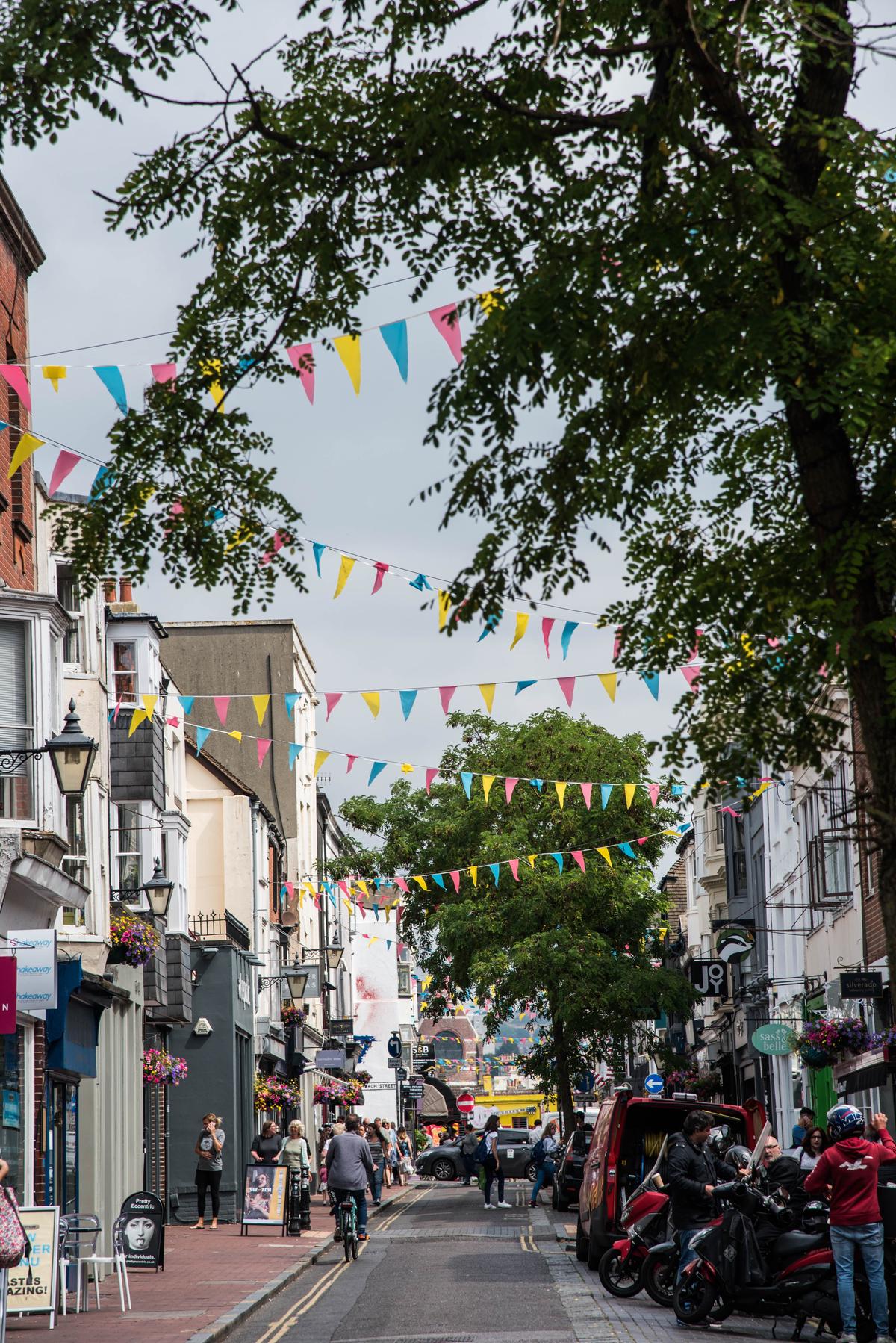
Real-world AWS CLI Scripting Scenarios
Setting up AWS CLI
Before you can start scripting with AWS CLI, you need to install it on your computer. AWS provides a comprehensive guide to the installation process here: https://aws.amazon.com/cli/. After it’s installed, configure AWS CLI by running ‘aws configure’ in your terminal. You’ll need your AWS Access Key ID, Secret Access Key, default region name, and the default output format.
Writing Your First Script
Your first script might be something simple, like listing all of your S3 buckets. Here’s how you can do that:
#!/bin/bash
aws s3 ls
Save the script as a .sh
file and run it by typing bash your-script-name.sh
in the terminal.
Scripting for AWS Automation
Let’s automate the process of starting and stopping an EC2 instance. Do note that for this script, you will need the instance ID which can be found in the EC2 console in your AWS dashboard.
Here is a bash script to stop an instance:
#!/bin/bash
aws ec2 stop-instances --instance-ids YOUR_INSTANCE_ID
And a similar one to start an instance:
#!/bin/bash
aws ec2 start-instances --instance-ids YOUR_INSTANCE_ID
These are very simple AWS CLI scripts and act as a good starting point for more complex automation.
Useful AWS CLI Scripting Scenarios
-
Monitoring: Writing a script to grab Cloudwatch metrics can help you automatically monitor resource use and troubleshoot any issues.
-
Deployment: AWS CLI can automate application deployment and environment configuration.
-
Backup: AWS CLI makes it easy to backup files to S3.
Here’s a script that copies a directory and all its contents to an S3 bucket:
#!/bin/bash
aws s3 cp /path/to/directory s3://bucket-name --recursive
Before writing these scripts, always make sure you have carefully considered the necessary AWS permissions, to prevent unauthorized access and secure your resources.
Conclusion
There’s a lot of potential with AWS CLI for scripting and automation. Numerous further resources from AWS provide examples and deeper dives into how you can use scripts to simplify and automate your AWS tasks.
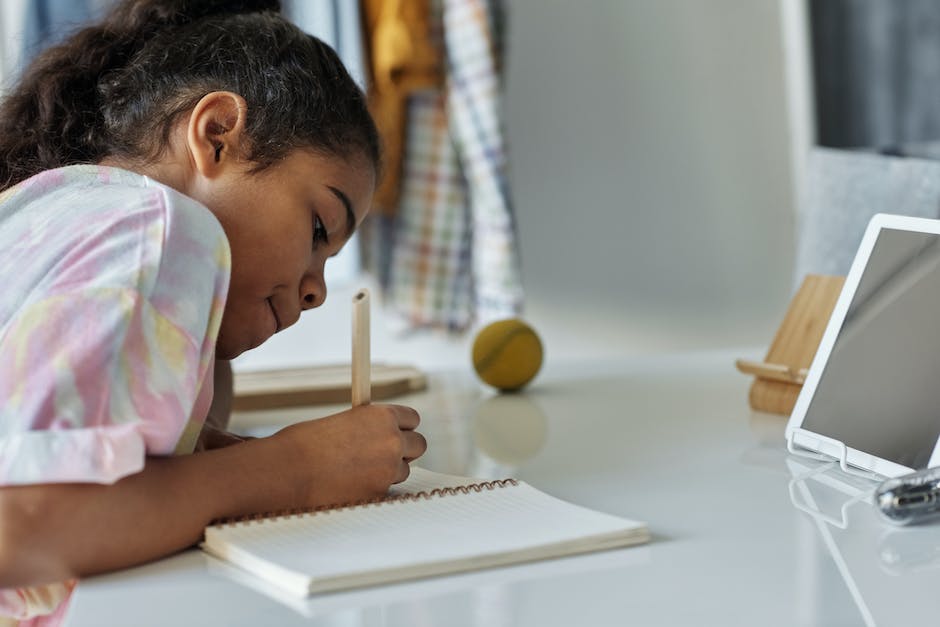
As we wrap up, it’s important to comprehend that the journey of learning AWS CLI scripting is a continuous one filled with new discoveries and possibilities at every turn. The knowledge gained on leveraging this tool will not just make tasks easier, but also open doors to larger avenues of creativity and problem-solving in cloud computing. The ability to design and implement purposeful, well-optimized scripts will allow you to harness the countless benefits of automation in AWS. So with a solid foundation in AWS CLI basics and advanced scripting laid, we look forward to seeing how you bring your newly acquired skills to life in unique and transformative ways.
Discover Writio, the innovative AI content writer that crafts impeccable articles tailored to your preferences. This exceptional piece was composed by Writio.